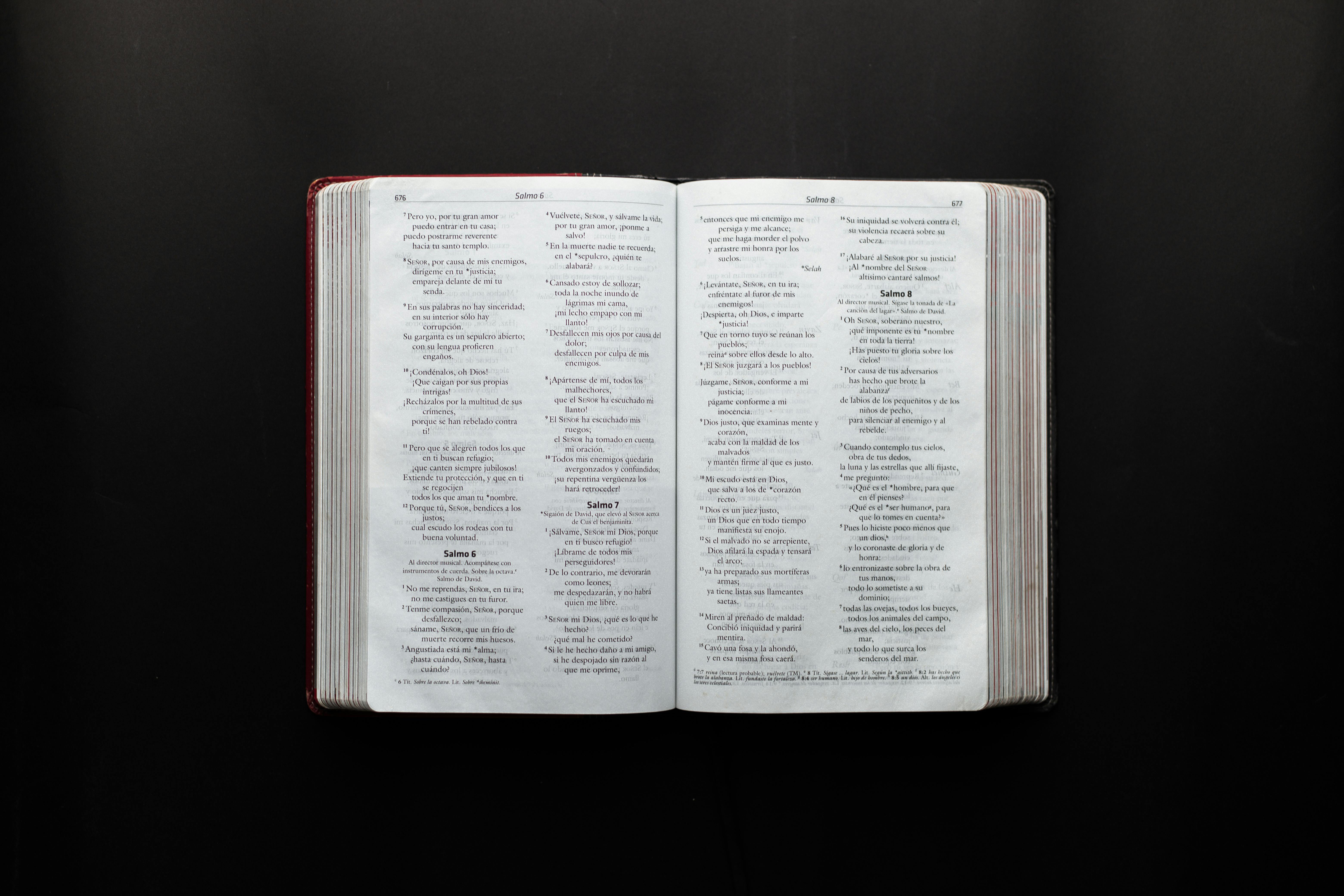
Essential Guide to Using GDB Effectively for Debugging in 2025
Debugging is an essential skill for developers, and GDB (GNU Debugger) stands out as one of the most powerful debugging tools available today. In this comprehensive guide, we will explore the intricacies of GDB, covering key commands and features that will enhance your debugging experience in 2025. Understanding how to utilize GDB can significantly improve your productivity when troubleshooting complex code, particularly for C/C++ applications.
Whether you are a beginner looking to master GDB commands or an advanced user seeking tips on reverse debugging and process tracking, this article is tailored for you. We’ll cover how to install GDB, set breakpoints, navigate through code effectively, and examine memory, among other essential techniques. By the end of this guide, you'll be equipped with practical knowledge to debug effectively using GDB.
Let’s take a closer look at the roadmap for this article:
- Overview of GDB and its installation
- GDB commands for beginners
- Advanced GDB features and techniques
- Strategies for debugging multi-threaded applications
- Utilizing GDB for remote debugging and automation
- Best practices and common pitfalls
Get ready to debug like a pro with the following insights on GDB!
GDB Overview and Installation for Beginners
To begin debugging with GDB, you first need to understand what GDB is and how to set it up in your environment. GDB is a versatile command-line debugger that allows developers to monitor and control program execution. It provides insights into the behavior of applications, which is crucial for identifying and fixing errors.
1. **GDB Installation**: Installing GDB is straightforward. On most Linux distributions, you can use the package manager. For example:
sudo apt install gdb
On macOS, you can install GDB via Homebrew:
brew install gdb
For Windows, GDB comes with the MinGW development environment, which you can download and install from the official MinGW website.
2. **Setting Up Your Environment**: After installing GDB, ensure that your build process includes debugging symbols. You can achieve this by compiling your C/C++ programs with the `-g` flag:
gcc -g myprogram.c -o myprogram
With your installation complete, you're now ready to dive into using GDB!
Mastering GDB Commands for Common Tasks
Once GDB is installed, knowing the right commands to navigate through your code is vital. This section will cover fundamental commands and their practical applications.
Basic Commands and Navigation
Understanding basic GDB commands is essential for effective debugging. Here are some key commands to get you started:
- **Starting a Program**: Use the `run` command to start your program within GDB:
(gdb) run
- **Setting Breakpoints**: To pause program execution at a specific line or function, use:
(gdb) break main
- **Stepping Through Code**: The `step` command allows you to execute the program line-by-line while the `next` command executes the next line without stepping into functions:
(gdb) step
(gdb) next
- **Checking Variables**: Inspect the current value of variables using:
(gdb) print variable_name
These commands form the basis for a clear understanding of how to navigate your code while debugging.
Utilizing Breakpoints and Watchpoints
Breakpoints and watchpoints are critical for tracking down issues in your code. Breakpoints stop execution at pre-defined locations, while watchpoints monitor variable values:
- **Conditional Breakpoints**: You can set up breakpoints that only activate when certain conditions are met, using:
(gdb) break my_function if x == 5
- **Watchpoints**: Use watchpoints to monitor changes to variable values:
(gdb) watch my_variable
These tactics allow you to narrow down your debugging efforts efficiently, improving the debugging workflow.
Advanced GDB Techniques for Experienced Users
As you become more comfortable with GDB, explore advanced features that can further enhance your debugging process.
Reverse Debugging and Catching System Calls
One of the standout features of GDB is its capability for reverse debugging, which allows you to step back through previous states of your application:
- **Enabling Reverse Debugging**: Use the `target record` command to start recording program states:
(gdb) target record
Then to step backwards, utilize:
(gdb) reverse-continue
- **Catching System Calls**: You can monitor system calls that your application makes, which can help diagnose issues related to system resources:
(gdb) catch syscall
These advanced techniques are invaluable for dealing with complex application behavior.
Debugging Multi-Threaded Applications with GDB
Debugging multi-threaded applications presents unique challenges, but GDB provides various features to manage these efficiently.
Managing Threads in GDB
When working with multi-threaded applications, understanding how to control threads in GDB is vital:
- **Listing Threads**: Use the command `info threads` to display all active threads:
(gdb) info threads
- **Switching Between Threads**: To switch the context to a specific thread, use:
(gdb) thread thread_id
Mastering these commands enables effective management of concurrency issues during debugging.
Using GDB for Remote Debugging and Automation
Remote debugging capabilities open up new possibilities for troubleshooting issues on different machines, while automation streamlines your debugging processes.
Setting Up Remote Debugging with GDB
To debug applications running on remote systems, GDB supports setting up remote debugging sessions:
- **Starting a Remote Session**: Use the `target remote` command, providing the IP address and port:
(gdb) target remote hostname:port
This allows you to connect to an application running on a remote server for detailed inspection.
Automating Debugging Tasks with GDB Scripts
You can automate repetitive debugging tasks using GDB scripts. Scripting enables you to write custom commands and sequences that reduce manual input:
- **Creating a Script**: Write your commands in a text file, then load them in GDB with:
(gdb) source my_script.gdb
Using scripts can significantly speed up the debugging process, especially when working on large projects.
Best Practices for Effective Debugging with GDB
Finally, let's discuss best practices that will enhance your overall debugging efficiency and effectiveness.
Common Pitfalls to Avoid
While using GDB, there are some common mistakes to avoid:
- **Neglecting Debug Symbols**: Always compile code with `-g` to include debug symbols; without them, GDB won't provide useful insights.
- **Ignoring Documentation**: GDB's extensive documentation is a valuable resource—be sure to consult it for commands and options.
Expert Recommendations for Enhanced Debugging
Consider these tips from experienced developers:
- **Regularly Update GDB**: Keep GDB updated to benefit from the latest features and fixes.
- **Experiment with Commands**: Familiarize yourself with the GDB user manual and practice using different commands during regular coding tasks.
By implementing these best practices, you can leverage GDB's full potential for effective debugging.
Q&A Section
1. What is GDB and why should I use it for debugging?
GDB is the GNU Debugger, a powerful tool for debugging applications written in languages like C and C++. It allows developers to see what's happening in a program while it executes or what it was doing at the moment it crashed.
2. How do I set breakpoints in GDB?
You can set breakpoints using the `break` command followed by the line number or function name where you want the program to stop executing.
3. Can I debug multi-threaded applications with GDB?
Yes, GDB has specific commands for managing and inspecting threads in multi-threaded applications, allowing you to analyze issues that arise in such environments.
4. How can I save the state of my debugger session?
GDB allows you to save the state of your debugging session by using the `gdb command history` to recall previous commands.
5. What are the benefits of using GDB scripts?
GDB scripts automate commonly used sequences of commands, saving time during extensive debugging sessions and reducing the chance of errors during manual input.
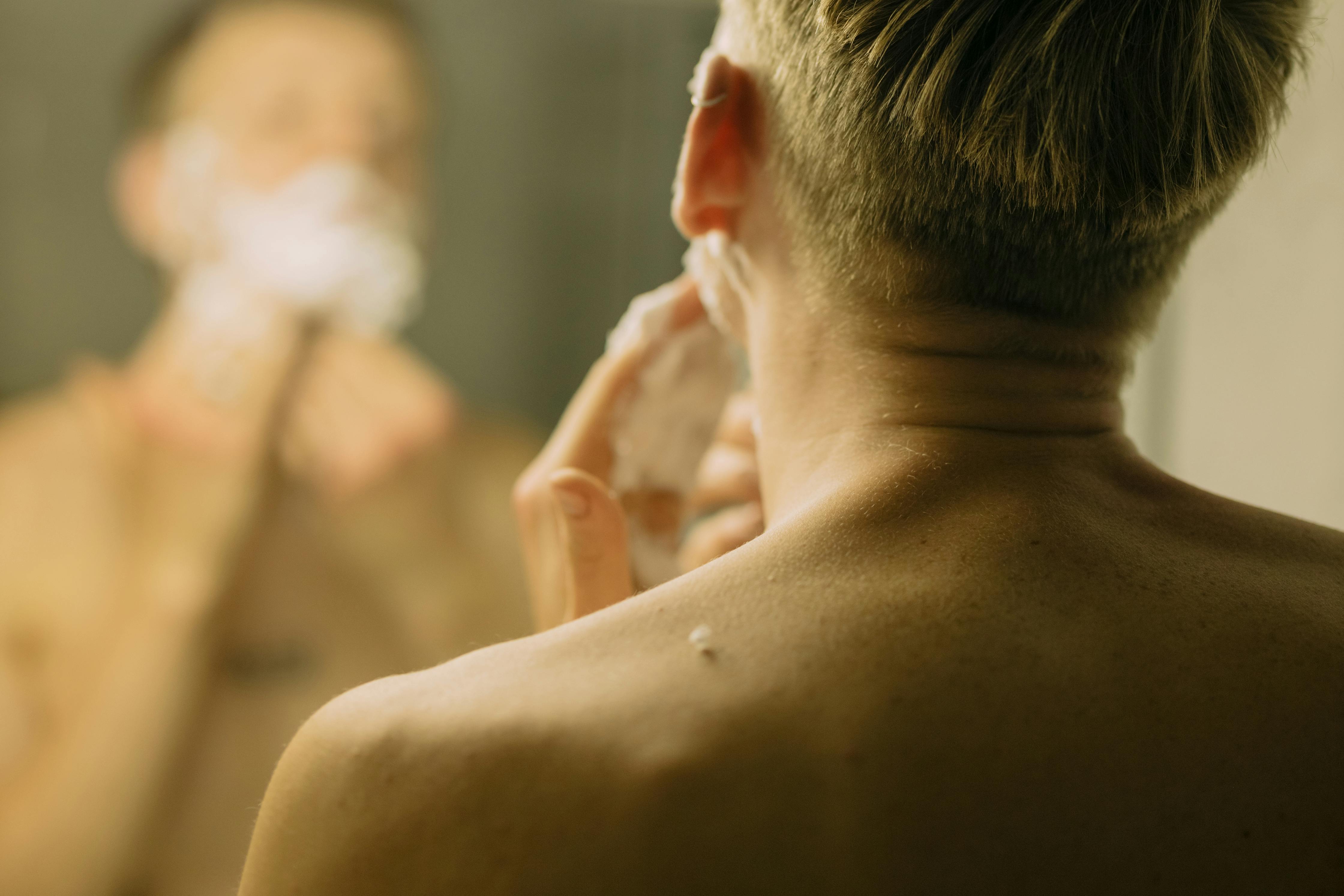
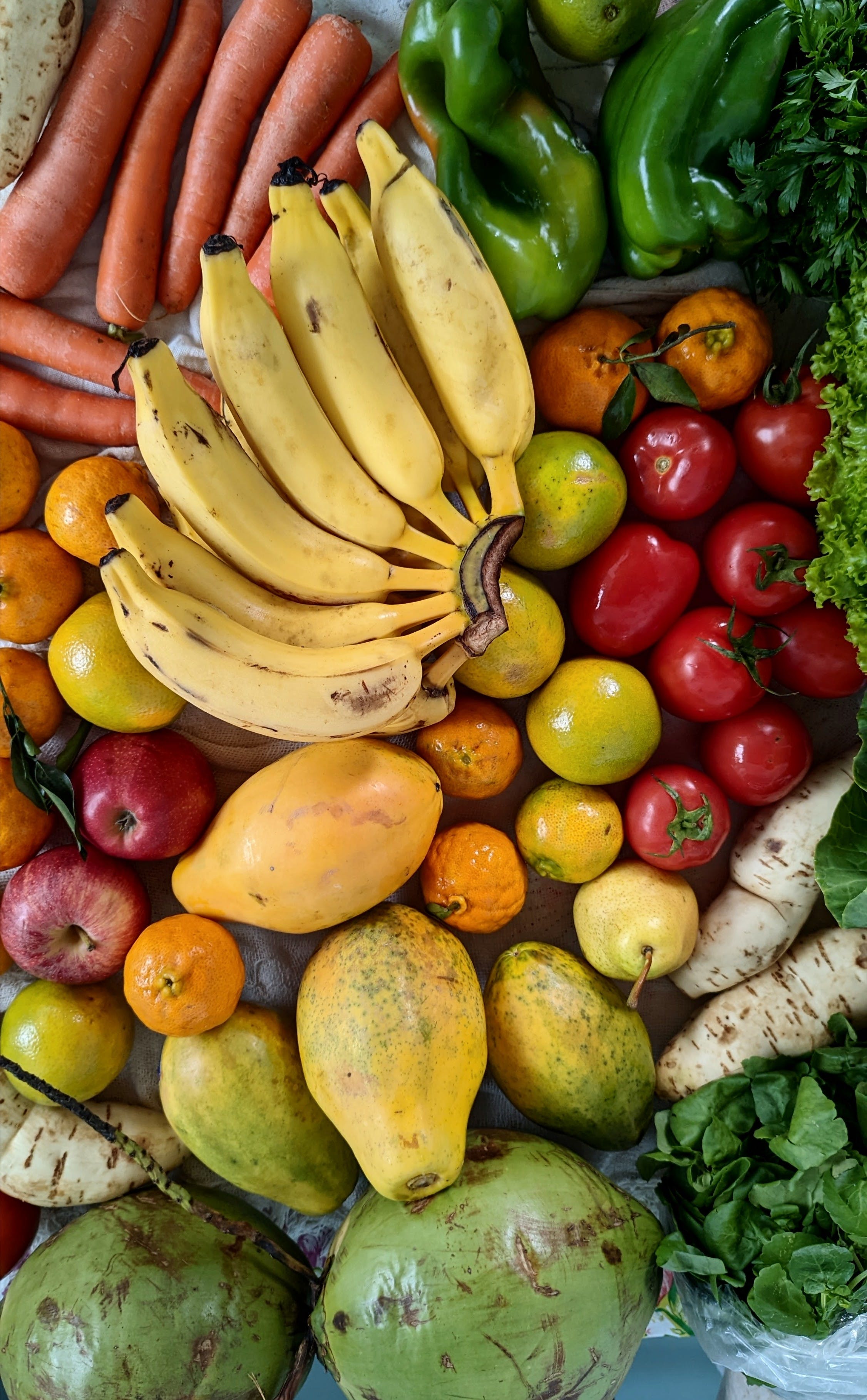